Getting Started#
This tutorial is available on youtube
Verify Installation#
Open a new project
- Make sure Plugin content is visible
Content Drawer / Settings / Show Engine Content [X]
Content Drawer / Settings / Show Plugins Content [X]
- Load Example Map
Plugins / GKFogOfWarContent / Example / FogOfWar
- Set Game Mode Override
WorldSettings / GameMode Override / GKFoW_GameMode
Create your own#
Initial Setup#
- Create a new Game State from
GKFoW_GameState
(inherits fromAGKFogOfWarGameState
) Update the team information if need be
AGKFogOfWarGameState > Teams > Fog Of War Teams
- Create a new Game State from
- Create a new Game Mode from GKFoW_GameMode (inherits from
AGKFogOfWarGameMode
) Inside GameMode set the game state to the newly created game state
Note
The game mode is used to initialize the Fog Of War before the game starts. You can override
AssignTeamFor
to customize how teams are assigned to player controller- Create a new Game Mode from GKFoW_GameMode (inherits from
- Set the Game Mode Override
WorldSettings / GameMode Override / GKFoW_GameMode
Tag the player start with the team name the player should be starting as
Add a
AGKFogOfWarVolume
to the scene and make it cover the entire mapNote
Only one volume can be created per map
Warning
The fog of war texture size should not be above 2048x2048 px (8192 / 4) IF GPU/CPU upscaling is enabled (it is by default)
Add obstacles#
- Create a new Pawn
Add a
UGKFogOfWarComponent
UGKFogOfWarComponent > GivesVision [ ]
UGKFogOfWarComponent > BlocksVision [X]
Add static mesh
Place Pawn on the map
Note
You will want to align the obstacles to the fog of war grid to make the bounds match as closely as possible. See Actor snapping.
Add Landscape#
- Create a landscape
Add bumps
- Add the landscape to the Fog of War Volume
Fog Of War Volume / Strategy / Landscape
Make sure the Fog of war is below the landscape
Make sure the Navigation Bound Volume includes the landscape
Custom collision Shapes#
Open an Obstacle Pawn
Add Box/Sphere/Cylinder Collision Shape
Make sure the shape covers the area that blocks visions
- Implement the
GKFogOfWarAgentInterface
Class Settings / Interfaces / Add / GKFogOfWarAgentInterfaces
Implement GetFogOfWarComponent by returning the component
Implement GetFogOfWarCollisionShape by returning the shape
- Implement the
Note
Only rectangle, yaw rotated rectangle and circle obstacles are supported.
Update Cylinder Obstacles#
- Open an Obstacle Pawn
Add Box/Sphere/Cylinder Collision Shape
Make sure the shape covers the area that blocks visions
Class Settings / Interfaces / Add / GKFogOfWarInterfaces
Implement GetFogOfWarComponent by returning the component
Implement GetFogOfWarCollisionShape by returning the shape
Player Starts#
- Create a Second PlayerStart
Change the tag to the enemy team (BadGuys)
- Change the Network mode to Clients
Editor / Change Play Mode / Net Mode / Client
- Change the number of players to 2
Editor / Change Play Mode / Number of Players / 2
Note
You should enable client side navigation for clients to move correctly.
Project Settings / Engine / Navigation System / Allow Client Side Navigation [X]
Debugging#
- Navigate to
Plugins / GKFogOfWar Content / Debug / GKFoW_DecalView
Drag and drop the decal on the map
Scale it to cover the map
Enable debug mode on the Fog of War Volume
Disable auto-exposure#
The fog of war will darken your screen, by default UE has auto exposure enabled which can create a distracting effect when large area are revealed.
Drag a drop a post process volume
- Disable Auto exposure
Post Process Volume / Exposure / Min Brightness / 1
Post Process Volume / Exposure / Max Brightness / 1
- Make the Post Process Volume unbounded
Post Process Volume / Volume Settings / Infinite Extent [X]
Details#
Classes#
AGKFogOfWarGameState
:implements
IGKFogOfWarGameStateInterface
provides a list of teams that will participate in the game
AGKFogOfWarGameMode
:extends AGameMode
expose new blueprint event
AssignTeamFor
used to assign a team to each player controller
AGKFogOfWarPlayerController
:extends the base PlayerController to make it belong a given team; it handles dynamic team change.
AGKFogOfWarCharacter
:extends the base Character
implements fog of war conditional replication, it is only replicated to enemies if it is seen by them.
AGKFogOfWarPlayerState
:extends
APlayerState
expose a new attribute
AGKFogOfWarPrivatePlayerState
which is only replicated to allies.
AGKFogOfWarVolume
:Draws the fog of war periodically
UGKFogOfWarComponent
:Register itself to the Fog of War volume
Specify how the actor fog of war should behave (blocks light, provide vision, vision radius)
UGKFogOfWarStrategy
:Implements how the fog is drawn
UGKTransformerStrategyCanvas
:Implements material based transformation on the vision texture
this is used to implement upscaling and exploration (blending of previous & current vision)
Initialization Flow#
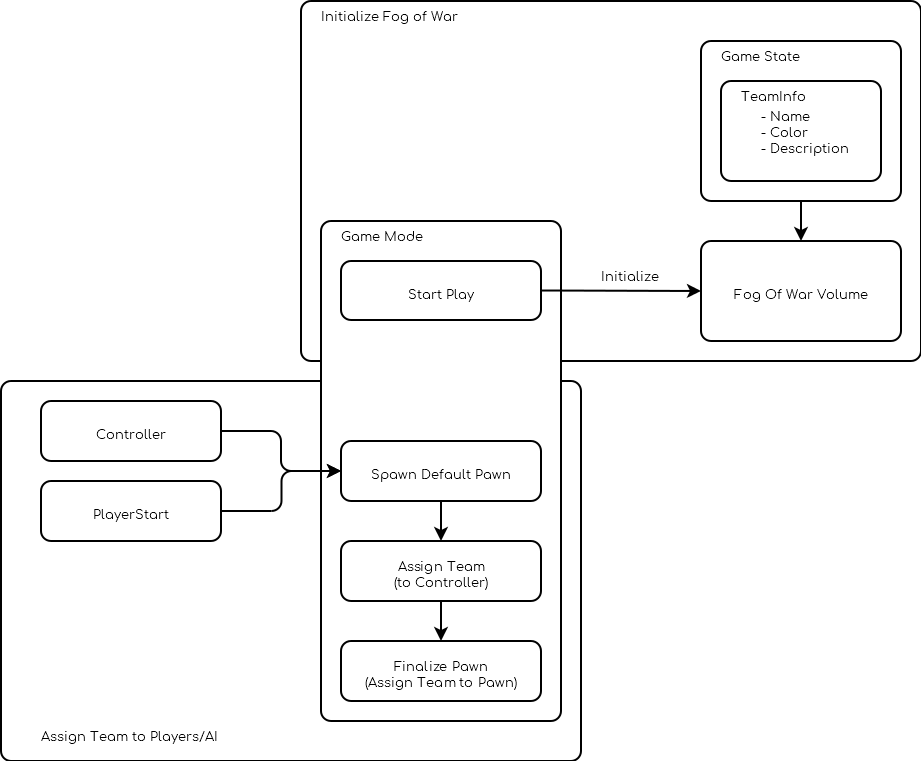
Replication Flow#
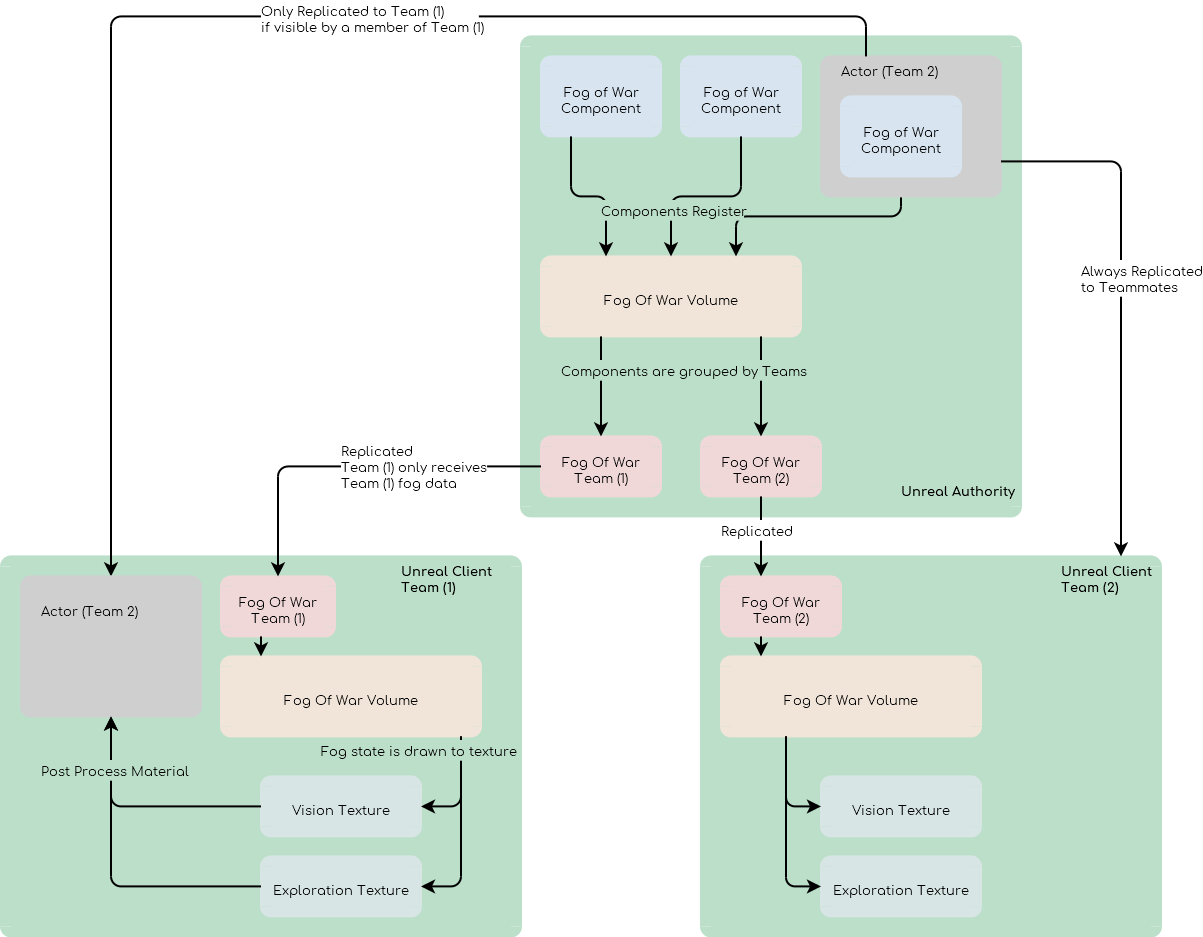