Getting Started#
This tutorial is available on youtube
Verify Installation#
Open a new project
- Make sure Plugin content is visible
Content Drawer / Settings / Show Engine Content [X]
Content Drawer / Settings / Show Plugins Content [X]
- Load Example Map
Plugins / GKAbility Content / Example / GKAbility
- Set Game Mode Override
WorldSettings / GameMode Override / GKAbility_GameMode
Setup#
- Create inputs use an EnhancedInputContext
Gamekit provided a few predefine action you can reuse including ConfirmTarget & CancelTarget input/Action
Create a new enum with the same action/input names (see
EGK_MOBA_AbilityInputID
as an example)Create a Character from
GKCharacterBase
Extend the On Setup Player Input Component event, use to register your input to the ability system component (See
AGKCharacterBase::BindAbilityActivationFromInputEnum()
)Warning
FGKGameplayAbilityInputBinds::CancelCommand
andFGKGameplayAbilityInputBinds::ConfirmCommand
are the named defined in the input’s project setting NOT the enum name.You are ready to grant abilities using
AGKCharacterBase::GrantAbility()
In Gamekit abilities are granted to a character for a given slot, each ability populates a slot. Slots can then be assigned an input. Gamekit defines 22 default slots (6 Skills, 8 Items, 7 Basic Abilities). Under the hood slots are simple integer, users can add as many slots as they want.Note
Granting ablities can only be done by the authority
During Gameplay, you can press inputs and the abilities will activate.
Example#
‘Legacy’ Player Input
Enhanced Player Input
Creating Abilities#
Create a new DataTable using the GKAbilityStatic struct
Insert a row, give the row a name (the row name is used to generate the ability asset)
Click the generate button
Warning
Python Editor Scripting plugin needs to be enabled & configured
Creating GameplayEffect#
For simple gameplay effect that modify attributes you should configure the magniture to be set by caller using the tag “Ability.Magnitude”. By default, UGKGameplayAbility will populate the data from the FGKAbilityStatic data.
Define Abilities#
Abilities are define inside a json file.
The list of customizable properties can be found here FGKAbilityStatic
.
Gameplay designer can create new abilities by simply adding new abilities inside the ability data file.
To create the new abilities in unreal engine you need to generate them using a python script.
Enable python scripting for UnrealEngine
Add
Gamekit/Script
to your python pathGo to
Gamekit/Content/Editor/Ability_Generator
right click on it andRun Editor Utility Widget
. A widget should pop up with a bit “Generate Default Abilities” buttonClick on the button, this will generate all the missing abilities
Example#
{
"Name": "Fireball",
"AbilityKind": "Skill",
"LocalName": "NSLOCTEXT(\"[0A85C43C484A243EF7B6B7B642AD2ACB]\", \"EC9BA0DD417F0C07C07E3DBB00F88785\", \"Fire Ball\")",
"LocalDescription": "NSLOCTEXT(\"[0A85C43C484A243EF7B6B7B642AD2ACB]\", \"9CB2744E447BF19F751B929142E6F484\", \"Launch a roaring ball of fire in a straight line\")",
"Icon": "Texture2D'/Gamekit/Textures/IconsSkills/fireball-red-1.fireball-red-1'",
"MaxLevel": 4,
"Duration": 0,
"AbilityEffects":
{
},
"Cost":
{
"Attribute":
{
"AttributeName": "Mana",
"Attribute": "/Script/Gamekit.GKAttributeSet:Mana",
"AttributeOwner": "Class'/Script/Gamekit.GKAttributeSet'"
},
"Value": [ 10, 9, 8, 7 ]
},
"Cooldown": [ 0.5, 9, 8, 5 ],
"Price": 0,
"MaxStack": 1,
"AreaOfEffect": 50,
"CastMaxRange": 500,
"CastMinRange": 0,
"AbilityBehavior": "PointTarget",
"AbilityTargetActorClass": "BlueprintGeneratedClass'/Game/Abilities/TargetActors/ControllerTrace.ControllerTrace_C'",
"TargetObjectTypes": [],
"CastTime": 0.20000000298023224,
"ChannelTime": 0,
"AbilityAnimation": "Attack",
"ProjectileActorClass": "BlueprintGeneratedClass'/Game/Abilities/Projectiles/BP_GA_Projectile.BP_GA_Projectile_C'",
"ProjectileSpeed": 1000,
"ProjectileBehavior": "Directional",
"ProjectileRange": 1600,
"AOEActorClass": "None"
}
Ability Kinds#
MaxLevel = HeroLevel / 2 UltimateMaxLevel = HeroLevel / 6
- Standard Ability
4 Levels, Every 2 level character increase their maximum basic level ability by 1
- Ultimate Ability
3 Levels, every 6 level
- Abilities with charges
Ability consume charges, charges are given by an effect periodically
- Passive abilities
Ability simply provide a permanent effect that can be temporarlly disabled with breaks
Linked Abilities [TODO]
Sub abilities [TODO]
Autocast abilities [TODO]
Modifier abilities [TODO]
Overview#
Ability DataTable Initialization#
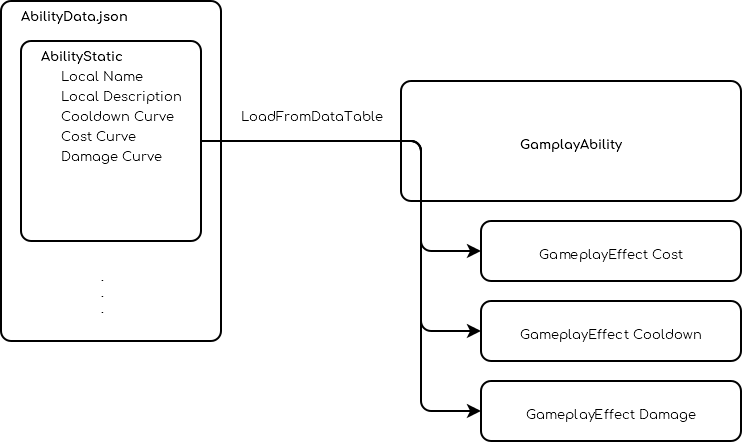
Ability Activation Flow#
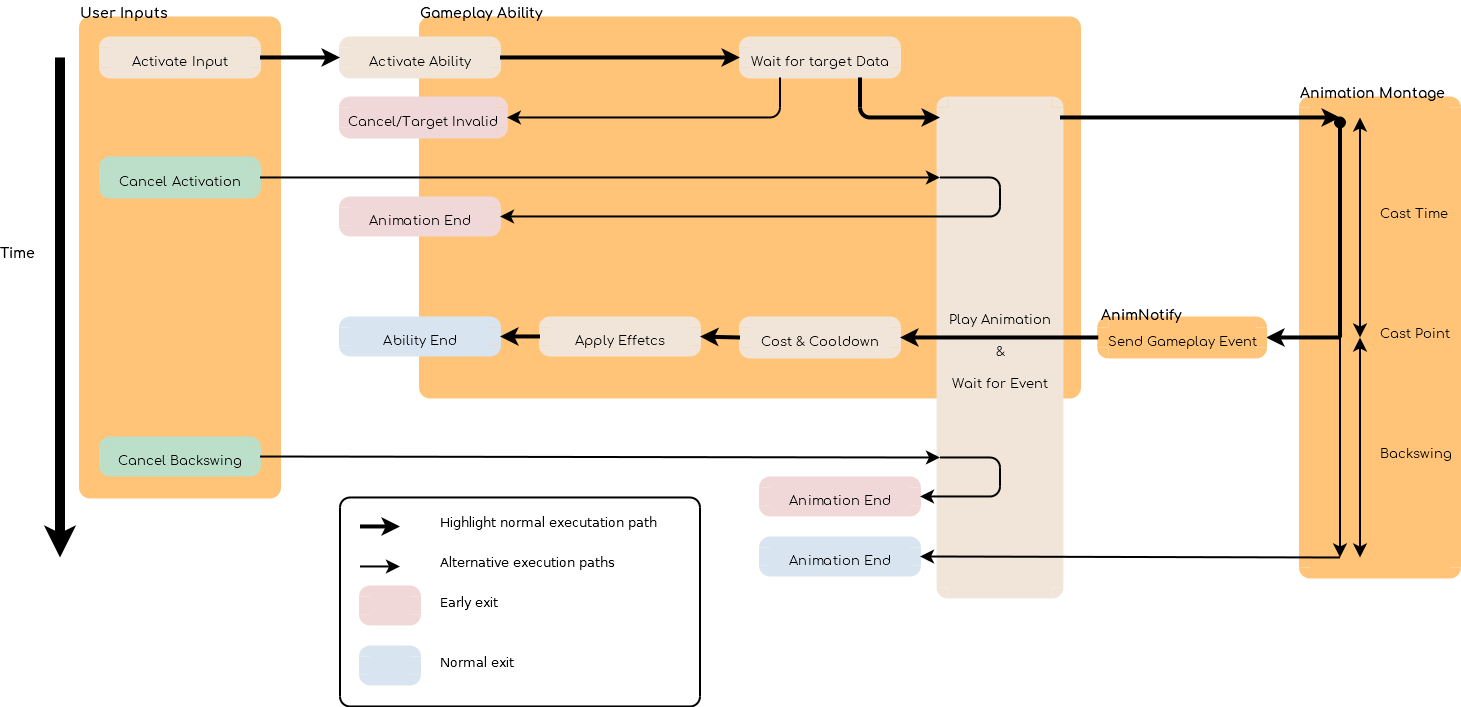
Ability Replication Flow#
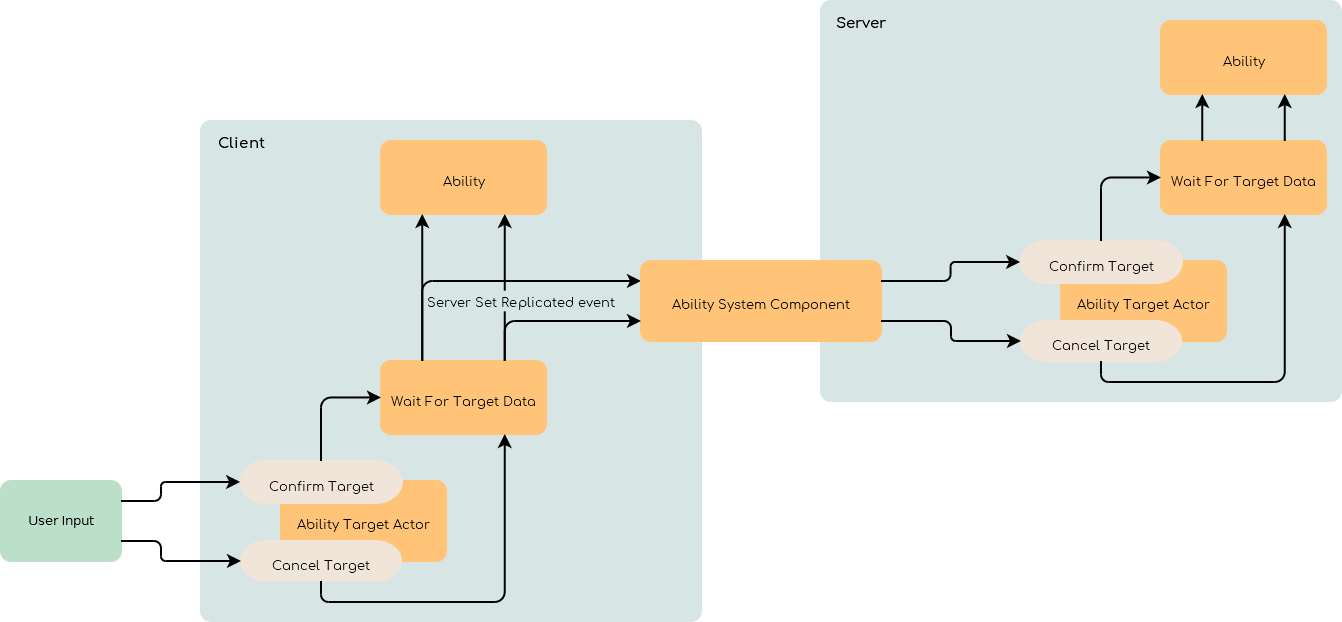
Animations#
To make abilities generic, the animations montage themselves are not specified.
Instead an animation kind is set (Channelling, Attack, Cast, etc..) which is a single enumerator
EGK_AbilityAnimation
representing the kind of annimation an ability can use.
The animation montage are specified by the Character itself through its FGKAnimationSet
(inside FGKUnitStatic
)
which our ability can sample from.
When an ability is activated the FGKAnimationSet
is fectched from the character to the ability
and the right animation is then played. This enable us to use the same ability for different
characters which can have different animation as well.
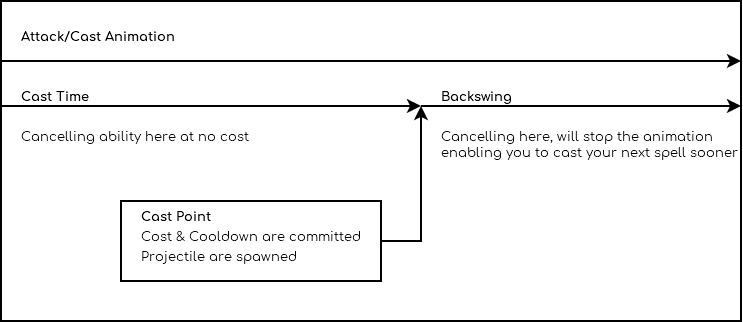
Ability Queue#
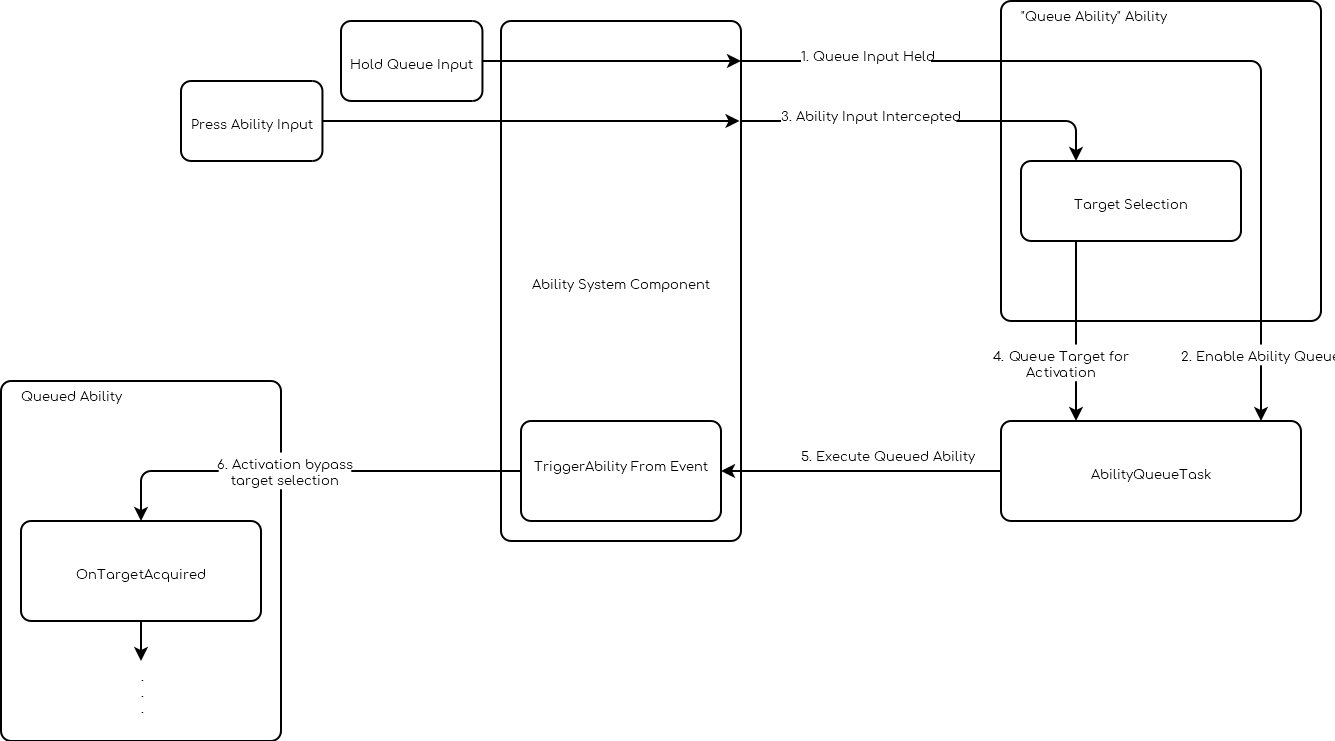
User Interface#
UI & Ability State#
The easiest way to bind the ability is to leverage the UGKAbilityWidget
coupled with UGKAbilitySystemEventManager
.
Instantiate a new
UGKAbilitySystemEventManager
, callSetupDelegates
to start listenning to events from theUAbilitySystemComponent
Register
UGKAbilityWidget
to the event manager usingAddAbilityWidget
thenSetupAbilityWidgetDelegates
to start receiving events.
Note
AddAbilityWidget
expects an interger as ID, by default it uses FGameplayAbilitySpec.InputID
.
The behaviour can be overiden by implementing GetWidgetIDFromAbilitySpecHandle
and GetWidgetIDFromAbilitySpec
.
Currently InputID
was used over FGameplayAbilitySpecHandle
because it allows us to create Ability widget before the
ability is even granted.
Builtin Abilities#
Base Ability: Basic ability with activation logic
Cancel Ability: Cancel all the current abilities
Move Ability: Move to destination or Actor
Base Skill: Ability that is disabled when silenced
- Base Item: Ability that is disabled when muted
with an optional item slot (Gloves, ring, etc…)
Builtin Effects#
Cooldown: block the casting of an ability for a given amount of time
Death: Remove all active effects and set health to zero
Dispel: Remove all debuffs and disables
Heal: Heal ocne
HealOvertime: Heals over time
Damage: Deals damage once
DamageOvertime: Deals damage overtime
Immunity: Grant immunity against all debuffs and disables
IncreaseHealth: Increase health
ManaCost: Remove mana from character
MoveHaste: Increase movement speed
Root: Prevent the character from moving
Silence: Prevent the character from casting spells
Stun: Completely disables the character
Builtin Attribute Set#
Health
Mana
Experience
Gold
Extending Gameplay Ability system#
Add delegates to the component
AI#
You can use
TriggerAbilityFromGameplayEvent
to trigger abilities without going though target selection
Ability Queue#
The ability queue is build by the AbilityQueue Ability, it is its own ability. It is only available on the client
Classes#
Relevant classes:
UGKGameplayAbility
: basic Gameplay Ability for skills and items, implements a few top down casting optionsFGKAbilityStatic
: configurable settings for abilitiesAGKAbilityTarget_Actor
: used to select a target for a given abilityUGKAbilitySystemComponent
UGKCastPointAnimNotify
: used to notify the ability when the animation reached a given pointUGKAttributeSet
: defines a set of attributes for characters (Health, Mana, Stamina, etc…)UGKAbilityWidget
: defines a basic interface for widget representing an ability state